The export functionality to the document on the server is extremely useful for converting HTML content to MS word document and download it as a.docx file. The MS word document can be quickly generated with HTML content via PHP.
Converting HTML to MS Word Document used primarily in the web application to generate.doc/.docx file with dynamic HTML data. The most popular file format is the Microsoft word document for exporting dynamic content offline. The JavaScript can be easily used to export HTML content to MS Word functionality. However, if you want to convert dynamic content to Doc, the interaction on the server-side is required. In this tutorial, we will show you step by step process to convert HTML to MS word file using PHP.
Step-1: Create html_to_doc.php file
In the html_to_doc.php file, we will write a custom class library code that helps to generate MS Word file and include the HTML content in Word document using PHP.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 |
<?php /** * Convert HTML to MS Word document * @name html_to_doc * @version 2.0 * @author TutorialsWebsite * @link https://www.tutorialswebsite.com */ class HTML_TO_DOC { var $docFile = ''; var $title = ''; var $htmlHead = ''; var $htmlBody = ''; /** * Constructor * * @return void */ function __construct(){ $this->title = ''; $this->htmlHead = ''; $this->htmlBody = ''; } /** * Set the document file name * * @param String $docfile */ function setDocFileName($docfile){ $this->docFile = $docfile; if(!preg_match("/\.doc$/i",$this->docFile) && !preg_match("/\.docx$/i",$this->docFile)){ $this->docFile .= '.doc'; } return; } /** * Set the document title * * @param String $title */ function setTitle($title){ $this->title = $title; } /** * Return header of MS Doc * * @return String */ function getHeader(){ $return = '<html xmlns:v="urn:schemas-microsoft-com:vml" xmlns:o="urn:schemas-microsoft-com:office:office" xmlns:w="urn:schemas-microsoft-com:office:word" xmlns="http://www.w3.org/TR/REC-html40"> <head> <meta http-equiv=Content-Type content="text/html; charset=utf-8"> <meta name=ProgId content=Word.Document> <meta name=Generator content="Microsoft Word 9"> <meta name=Originator content="Microsoft Word 9"> <!--[if !mso]> <style> v\:* {behavior:url(#default#VML);} o\:* {behavior:url(#default#VML);} w\:* {behavior:url(#default#VML);} .shape {behavior:url(#default#VML);} </style> <![endif]--> <title>'.$this->title.'</title> <!--[if gte mso 9]><xml> <w:WordDocument> <w:View>Print</w:View> <w:DoNotHyphenateCaps/> <w:PunctuationKerning/> <w:DrawingGridHorizontalSpacing>9.35 pt</w:DrawingGridHorizontalSpacing> <w:DrawingGridVerticalSpacing>9.35 pt</w:DrawingGridVerticalSpacing> </w:WordDocument> </xml><![endif]--> <style> <!-- /* Font Definitions */ @font-face {font-family:Verdana; panose-1:2 11 6 4 3 5 4 4 2 4; mso-font-charset:0; mso-generic-font-family:swiss; mso-font-pitch:variable; mso-font-signature:536871559 0 0 0 415 0;} /* Style Definitions */ p.MsoNormal, li.MsoNormal, div.MsoNormal {mso-style-parent:""; margin:0in; margin-bottom:.0001pt; mso-pagination:widow-orphan; font-size:7.5pt; mso-bidi-font-size:8.0pt; font-family:"Verdana"; mso-fareast-font-family:"Verdana";} p.small {mso-style-parent:""; margin:0in; margin-bottom:.0001pt; mso-pagination:widow-orphan; font-size:1.0pt; mso-bidi-font-size:1.0pt; font-family:"Verdana"; mso-fareast-font-family:"Verdana";} @page Section1 {size:8.5in 11.0in; margin:1.0in 1.25in 1.0in 1.25in; mso-header-margin:.5in; mso-footer-margin:.5in; mso-paper-source:0;} div.Section1 {page:Section1;} --> </style> <!--[if gte mso 9]><xml> <o:shapedefaults v:ext="edit" spidmax="1032"> <o:colormenu v:ext="edit" strokecolor="none"/> </o:shapedefaults></xml><![endif]--><!--[if gte mso 9]><xml> <o:shapelayout v:ext="edit"> <o:idmap v:ext="edit" data="1"/> </o:shapelayout></xml><![endif]--> '.$this->htmlHead.' </head> <body> '; return $return; } /** * Return Document footer * * @return String */ function getFotter(){ return "</body></html>"; } /** * Create The MS Word Document from given HTML * * @param String $html :: HTML Content or HTML File Name like path/to/html/file.html * @param String $file :: Document File Name * @param Boolean $download :: Wheather to download the file or save the file * @return boolean */ function createDoc($html, $file, $download = false){ if(is_file($html)){ $html = @file_get_contents($html); } $this->_parseHtml($html); $this->setDocFileName($file); $doc = $this->getHeader(); $doc .= $this->title; $doc .= $this->htmlBody; $doc .= $this->getFotter(); if($download){ @header("Cache-Control: ");// leave blank to avoid IE errors @header("Pragma: ");// leave blank to avoid IE errors @header("Content-type: application/octet-stream"); @header("Content-Disposition: attachment; filename=\"$this->docFile\""); echo $doc; return true; }else { return $this->write_file($this->docFile, $doc); } } /** * Parse the html and remove <head></head> part if present into html * * @param String $html * @return void * @access Private */ function _parseHtml($html){ $html = preg_replace("/<!DOCTYPE((.|\n)*?)>/ims", "", $html); $html = preg_replace("/<script((.|\n)*?)>((.|\n)*?)<\/script>/ims", "", $html); preg_match("/<head>((.|\n)*?)<\/head>/ims", $html, $matches); $head = !empty($matches[1])?$matches[1]:''; preg_match("/<title>((.|\n)*?)<\/title>/ims", $head, $matches); $this->title = !empty($matches[1])?$matches[1]:''; $html = preg_replace("/<head>((.|\n)*?)<\/head>/ims", "", $html); $head = preg_replace("/<title>((.|\n)*?)<\/title>/ims", "", $head); $head = preg_replace("/<\/?head>/ims", "", $head); $html = preg_replace("/<\/?body((.|\n)*?)>/ims", "", $html); $this->htmlHead = $head; $this->htmlBody = $html; return; } /** * Write the content in the file * * @param String $file :: File name to be save * @param String $content :: Content to be write * @param [Optional] String $mode :: Write Mode * @return void * @access boolean True on success else false */ function write_file($file, $content, $mode = "w"){ $fp = @fopen($file, $mode); if(!is_resource($fp)){ return false; } fwrite($fp, $content); fclose($fp); return true; } } |
In the above code,
- setDocFileName() – Set document file name.
- setTitle() – Set document title.
- getHeader() – Create header section of the document.
- getFotter() – Create footer section of the document.
- createDoc() – Create word document in .dcox format.
- _parseHtml() – Parse and filter HTML from source.
- write_file() – Insert content in the word file.
Step-2: Create index.php file
Copy and paste the following code in the index.php file. Here we will load the html_to_doc.php library and initialize the class. After this, we will specify custom HTML content to convert into Word document. Call the createDoc() function to convert HTML content to Word document.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
<?php include_once 'html_to_doc.php'; // Initialize class $htmltodoc = new HTML_TO_DOC(); $htmlContent = '<!Doctype html> <html> <head> <title>TutorialsWebsite</title> </head> <body> <h1>Hello World!</h1> <h1>Welcome to Tutorialswebsite.com</h1> <p>This document is created from HTML.</p> </body> </html>'; $htmltodoc->createDoc($htmlContent, "document"); ?> |
If you want to automatically download word file:
To download the word file, set the third parameter of the createDoc()
to TRUE.
2 3 4 |
$htmltodoc->createDoc($htmlContent, "document", 1); |
Generate Word document from HTML File:
You can convert the contents of the HTML file to a Word document by specifying the HTML file name.
2 3 4 |
$htmltodoc->createDoc("myfile.html", "document"); |
How to Convert an Image to PDF in CodeIgniter using FPDF Library
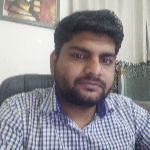
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co